Last updated on December 21st, 2023 at 07:35 am
Are you looking to develop Node.js project? In this comprehensive guide, we will take you through the step-by-step process of building a Node.js project. Whether you’re a beginner or an experienced developer, this article is designed to provide you with the knowledge and guidance you need. From setting up your development environment to writing and testing your code, we’ll cover it all.
Let’s get started!
What is Node.js?
Node.js is an open-source, server-side JavaScript runtime environment built on Chrome’s V8 JavaScript engine. It allows developers to execute JavaScript code outside the web browser, enabling server-side scripting and the development of high-performance network applications.
Node.js provides an event-driven, non-blocking I/O (input/output) model, which makes it lightweight and efficient for handling concurrent connections. This technology has gained popularity due to its scalability, speed, and ability to handle a large number of simultaneous requests.
Node.js is commonly used for developing web servers, real-time applications, APIs, microservices, and other server-side applications. Its extensive ecosystem of modules and packages makes it a versatile platform for building a wide range of applications.
Related: NPM vs PNPM: Which One Node JS Package Manager Should You Use?
Step By Step Process to Build A Node.js Project
The process of building a Node.js project involves a step-by-step approach to ensure a successful outcome. By following a systematic methodology, you can create robust and scalable applications using Node.js.
In this guide, we will take you through the step-by-step process of building a Node.js project, providing you with the necessary knowledge and guidance to embark on your development journey.
Install Node.js
Installing Node.js is an essential first step in building Node.js projects. Follow these detailed instructions to install Node.js on your machine:
Visit the Node.js website
Go to the official Node.js website at https://nodejs.org in your web browser.
Choose the appropriate installer
Node.js provides installers for various operating systems, including Windows, macOS, and Linux. On the Node.js website, the download button should automatically detect your operating system and recommend the appropriate installer. If it doesn’t, select the desired version for your operating system.
Download the installer
Click on the download button to start the download process. The installer file will be saved to your computer.
Run the installer
Locate the downloaded installer file and double-click it to run the installation. Depending on your operating system, you may need administrator privileges to proceed.
Windows installation steps
Windows Installer: If you downloaded the Windows Installer, an installation wizard will launch. Follow the instructions provided by the wizard, review the license agreement, select the destination folder, and choose any additional options you want to install (e.g., adding Node.js to the system PATH).
Windows Binary: If you downloaded the Windows Binary, extract the ZIP file to a desired location on your computer.
macOS installation steps
macOS Installer: If you downloaded the macOS Installer, an installation wizard will open. Follow the on-screen instructions, review the license agreement, and select the destination folder. You can also choose whether to install the npm package manager alongside Node.js.
macOS Binary: If you downloaded the macOS Binary, open the downloaded file and follow the instructions to complete the installation.
Linux installation steps
Linux Binaries: If you downloaded the Linux Binaries, extract the tarball to a desired location on your system.
Package Managers: Alternatively, you can install Node.js using package managers like apt, yum, or dnf, depending on your Linux distribution. Refer to the official Node.js documentation for instructions specific to your distribution.
Verify the installation
After the installation is complete, open a command-line interface (e.g., Command Prompt, Terminal) and enter the following command:
node -v
This command will display the version number of Node.js if the installation was successful.
Optional
Install a package manager (npm or yarn): Node.js comes with npm (Node Package Manager) by default. However, you can also choose to install yarn as an alternative package manager. To check if npm is installed, run the following command:
npm -v
To install yarn, refer to the official yarn documentation for the appropriate installation steps.
Congratulations! You have successfully installed Node.js on your machine. You can now start building Node.js projects and leveraging the capabilities of the Node.js runtime environment.
Initialize A New Project
Initializing a new project is an essential step when starting a Node.js project. It involves setting up the project structure, managing dependencies, and creating the necessary configuration files. Here’s a detailed explanation of how to initialize a new Node.js project:
Open your command-line interface
Launch your preferred command-line interface, such as the terminal on macOS/Linux or Command Prompt on Windows.
Navigate to the desired directory
Use the cd command to navigate to the directory where you want to create your Node.js project. For example, if you want to create the project in a folder named “my-node-project” on your desktop, you would run the command cd Desktop/my-node-project.
Initialize the project
Once you’re inside the desired directory, use the npm init command to initialize a new Node.js project. This command will prompt you to provide information about your project, such as the project name, version, description, entry point file, test command, and more.
Alternatively, if you want to skip the prompts and accept the default values, you can use the npm init -y command. This command automatically generates a package.json file with default settings.
Enter project details
As you run npm init, you will be prompted to enter various details about your project. Here’s an explanation of some of the commonly asked fields:
- Package Name: This is the name of your project. It should be unique and follow the naming conventions for npm packages.
- Version: Specify the initial version of your project. You can use semantic versioning (e.g., 1.0.0) to manage version updates.
- Description: Provide a brief description of your project, explaining its purpose and key features.
- Entry Point: Specify the main file that will be executed when running your Node.js application. By convention, it is often named index.js or server.js.
- Test Command: If you plan to write tests for your project, you can specify the command that will be used to run the tests.
- Author: Enter your name or the name of the project’s primary author.
- License: Choose an appropriate license for your project. Common options include MIT, Apache, and GNU.
Review and create package.json
After providing the necessary information, the npm init command will display a summary of the details you entered. Review the information to ensure its accuracy. If everything looks correct, press Enter to create the package.json file.
Manage dependencies
Once the project is initialized, you can start managing dependencies. Dependencies are external packages or libraries that your project relies on. They can be installed using the npm install command, followed by the package name.
For example, if you want to install the Express.js framework, you would run the command npm install express. This command fetches the package from the npm registry and installs it within your project’s node_modules directory. The installed package is then listed as a dependency in the package.json file.
Update package.json
As you install dependencies, the package.json file is automatically updated with the package names and their corresponding versions. It serves as a manifest file that defines your project’s dependencies, scripts, and other configuration options.
Additional project setup
Depending on your project’s requirements, you may need to create additional configuration files or directories. For example, you might create a .gitignore file to exclude certain files from version control or set up a config directory to store environment-specific configurations.
Initializing a new project sets the foundation for your Node.js development. It creates the necessary files and directories, establishes project metadata.
Create Project Structure
Creating a well-structured project is crucial for organizing your code and maintaining scalability in your Node.js project. Here are some key considerations for creating an effective project structure:
Root Directory
Start by creating a root directory for your project. Give it a meaningful name related to your project’s purpose. This directory will contain all the files and folders related to your Node.js project.
Source Code Directory (src)
Create a directory, commonly named “src” or “lib,” to store your project’s source code files. This directory will house your application’s logic, including route handlers, controllers, utility functions, and other core modules.
Routes Directory
Within the source code directory, create a “routes” directory. This directory will contain files responsible for handling different routes or endpoints in your application. Each file can represent a specific area or functionality of your application (e.g., user routes, authentication routes, API routes).
Models Directory
If your application interacts with databases or external services, create a “models” directory. This directory will house files responsible for defining data models, schema validations, and database-related operations. Each model file can represent a specific entity or data structure in your application (e.g., user model, product model).
Middleware Directory
For handling middleware functions, such as authentication, error handling, or request preprocessing, create a “middleware” directory. Store reusable middleware functions as separate files within this directory. This promotes modularity and helps maintain a clean codebase.
Config Directory
To manage configuration files for your project, create a “config” directory. This directory can include files like database configuration, environment variables, logging settings, or any other project-specific configurations. Centralizing these configurations simplifies maintenance and improves scalability.
Public Directory
If your project involves serving static files (e.g., HTML, CSS, images), create a “public” directory. Place your static assets in this directory, which will be accessible to users. It’s a good practice to separate static files from your application’s logic.
Tests Directory
Establish a “tests” directory to store your project’s test files. Organize your tests based on the corresponding source code files or modules they test. Use a testing framework, such as Mocha or Jest, to write and run your tests.
Additional Directories
Depending on the complexity of your project, you might need additional directories. For example, you can create a “controllers” directory within the source code directory to store files responsible for handling business logic, or a “views” directory for storing view templates in case you’re using a templating engine.
By creating a clear and organized project structure, you enhance code maintainability, collaboration, and overall project scalability. Following common conventions and industry best practices can make it easier for other developers to understand and contribute to your project. Remember to document your project’s structure and provide a README file that explains the purpose and usage of each directory and key files.
Install Dependencies
Installing dependencies is a crucial step in building a Node.js project as it allows you to incorporate external libraries, frameworks, and modules that enhance the functionality and efficiency of your application. Here’s a detailed explanation of the process:
Identifying Dependencies
Begin by identifying the specific dependencies your project requires. These can be third-party packages available on the npm (Node Package Manager) registry or modules developed by your team or the community. You can search for packages on the npm website (https://www.npmjs.com/) or use other resources like GitHub or community forums to discover relevant dependencies for your project.
Package Management with npm
npm is the default package manager for Node.js and comes bundled with the installation. It allows you to manage your project’s dependencies efficiently. To install a dependency, open your command-line interface, navigate to your project directory, and use the npm install <package-name> command.
Replace <package-name> with the name of the dependency you want to install. For example, to install the Express.js framework, you would run npm install express.
Package.json File
During the dependency installation process, npm automatically updates the package.json file in your project directory. This file acts as a manifest for your project, documenting its metadata, including the list of dependencies.
It also keeps track of the installed versions of each dependency. The package.json file is vital for consistency, reproducibility, and sharing your project with others.
Managing Dependencies Versions
By default, npm installs the latest stable version of a dependency. However, it’s essential to manage dependency versions to ensure compatibility and avoid unexpected issues. The package.json file provides a list of the installed dependencies and their respective version ranges.
You can define specific version numbers or specify version ranges using semantic versioning (e.g., “^2.0.0” for any version greater than or equal to 2.0.0). npm also offers commands like npm outdated to check for outdated dependencies and npm update to update dependencies within the defined version ranges.
Dependency Tree
Dependencies can have their own dependencies, forming a tree-like structure. npm automatically resolves and installs these transitive dependencies based on the package requirements.
The package-lock.json file, introduced in npm 5, maintains a complete and deterministic record of the dependency tree, ensuring consistent installations across different environments. It should be committed to version control along with the package.json file.
Working with DevDependencies
Besides regular dependencies, Node.js projects often have devDependencies. These are dependencies used during development but not required in the production environment. Examples include testing frameworks, build tools, and code quality plugins.
DevDependencies are installed separately using the –save-dev flag with the npm install command. They are also listed in the package.json file, but they won’t be installed when your project is deployed or distributed.
Semantic Versioning
npm follows semantic versioning (SemVer) for package versions. A version number consists of three digits: Major.Minor.Patch. Incrementing the major version indicates incompatible API changes, the minor version signifies added functionality in a backward-compatible manner, and the patch version represents bug fixes or minor updates without changing the API.
Understanding SemVer helps you manage and update dependencies while considering the impact on your project.
Alternative Package Managers
While npm is the default choice for package management in Node.js, alternative package managers like Yarn (https://yarnpkg.com/) are available. Yarn provides faster and more reliable dependency management, caching, and parallel downloads. It is compatible with the npm registry, allowing you to switch seamlessly between npm and Yarn.
By installing and managing dependencies effectively, you can leverage the vast Node.js
Write Your Code
When it comes to writing code for your Node.js project, there are several key aspects to consider. Here’s a detailed breakdown:
Entry Point File
Every Node.js project starts with an entry point file, often named server.js or index.js. This file serves as the starting point of your application and is responsible for setting up the server, defining routes, and managing the overall flow of the application.
Module System
Node.js follows the CommonJS module system, allowing you to break your code into modules for better organization and reusability. You can create modules by defining functions, objects, or classes and exporting them using the module.exports or exports keywords. Import these modules into other files using the require function.
Routing and Handling Requests
Node.js provides flexibility in handling HTTP requests. You can use the built-in http module or opt for popular frameworks like Express.js or Koa.js to simplify routing and request handling. Define routes that correspond to specific URLs and HTTP methods (GET, POST, PUT, DELETE) and specify the corresponding request handlers.
Business Logic
Implement the core functionality of your Node.js project within the appropriate modules. This can involve processing data, interacting with databases, making API calls, performing calculations, or any other operations specific to your project’s requirements. Break down complex tasks into smaller functions or methods for better maintainability.
Middleware
Middleware functions are an integral part of Node.js applications, particularly when using frameworks like Express.js. As an intermediary between the incoming request and the final route handler, middleware can perform tasks such as authentication, logging, error handling, or modifying request/response objects. Write and integrate middleware functions to enhance the functionality and behavior of your project.
Database Integration
Node.js projects often require interaction with databases. Whether you’re using SQL-based databases like MySQL or PostgreSQL or NoSQL databases like MongoDB, you’ll need to establish a connection, perform CRUD (Create, Read, Update, Delete) operations, and handle database queries efficiently. Utilize appropriate database drivers, ORMs (Object-Relational Mappers), or query builders based on your chosen database technology.
Error Handling
Node.js applications should handle errors gracefully to prevent crashes and provide meaningful feedback to users. Implement error handling mechanisms at various levels, such as global error handlers, route-specific error handlers, or middleware for error processing. Use try-catch blocks to catch and handle synchronous errors, and handle asynchronous errors using promises or the async/await syntax.
Testing
Ensure the reliability and correctness of your Node.js code by writing tests. Utilize testing frameworks like Mocha, Jest, or Chai to create test suites and test cases for different parts of your application. Cover edge cases, handle different input scenarios, and check for expected outcomes. Automate your tests to run them regularly, ensuring that your code continues to function as intended.
Logging and Debugging
Incorporate logging statements throughout your codebase to capture useful information about the application’s behavior. Log important events, errors, and data to aid in debugging and troubleshooting. Utilize Node.js debugging tools, such as the built-in debugger or popular IDEs’ debugging features, to step through code, inspect variables, and identify and resolve issues efficiently.
Code Documentation
Document your code to make it more readable and understandable for yourself and other developers. Add comments to explain complex logic, document function parameters and return values, and provide an overview of the purpose and functionality of your code. Additionally, consider generating API documentation using tools like JSDoc to automatically generate documentation based on code annotations.
By focusing on these aspects while writing your code, you can ensure a well-structured, maintainable, and robust Node.js project.
Test Your Project
Testing your Node.js project is a crucial step to ensure its functionality and reliability. By implementing tests, you can catch bugs and issues early on, saving time and effort in the long run. Use popular testing frameworks like Mocha, Jest, or Chai to write test cases for different modules and components of your project.
Cover various scenarios and edge cases to validate your code’s behavior. Running tests regularly helps maintain the stability of your application, giving you confidence in its performance. By prioritizing testing, you can deliver a high-quality Node.js project that meets user expectations and performs as intended.
Run and Debug
Running and debugging your Node.js project is crucial for ensuring its functionality and identifying and resolving issues. Here’s a detailed explanation of running and debugging your Node.js project:
Running Your Node.js Project:
To run your Node.js project, follow these steps:
- Open your command-line interface or terminal and navigate to the root directory of your project.
- Use the command node <entry-file> to start your Node.js application, where <entry-file> is the main file that serves as the entry point of your application (e.g., server.js).
- If everything is set up correctly, you should see the output or log messages indicating that your application has started running.
- Access your application by opening a web browser and entering the appropriate URL (e.g., http://localhost:3000 if you’re running a web server on port 3000).
Debugging Your Node.js Project:
Debugging helps identify and fix issues or unexpected behaviors in your Node.js project. Here are some techniques for debugging Node.js applications:
- Console Logging: Place console.log() statements at strategic points in your code to output specific values or log messages. These can help you understand the flow of execution and track variable values during runtime.
- Node.js Debugger: Node.js provides a built-in debugger that allows you to set breakpoints, inspect variables, and step through your code. To use the Node.js debugger, start your application with the –inspect flag (e.g., node –inspect <entry-file>). This will enable the debugging mode, and you can connect to it using a debugger tool like Chrome DevTools or Visual Studio Code.
- Debugging with Visual Studio Code: If you’re using Visual Studio Code as your code editor, it offers excellent debugging support for Node.js projects. Set breakpoints in your code, launch the debugger from the editor, and step through your code while inspecting variables and tracking the flow of execution.
- Logging Libraries: Consider using logging libraries like Winston or Bunyan, which provide advanced logging capabilities, including log levels, file logging, and more. These libraries can be useful for tracking application behavior and identifying issues.
- Error Handling: Implement proper error handling in your Node.js project to catch and handle exceptions. Use try-catch blocks to capture errors and log relevant information for debugging purposes.
- Debugging Tools: Various debugging tools and extensions are available for Node.js development. These tools offer advanced features like real-time code reloading, automatic restart on code changes, and more. Examples include Nodemon, Node Inspector, and ndb.
Remember to remove or disable any debugging-related code or settings in your production environment to avoid unnecessary overhead.
By effectively running and debugging your Node.js project, you can ensure its smooth operation, identify and fix bugs, and deliver a high-quality application to your users.
Document Your Project
Documenting your project is a crucial step in the development process. It involves creating clear and concise documentation to aid understanding and collaboration among developers. Documenting your project includes adding comments to your code, creating README files, and generating API documentation.
These documents provide essential information about the project’s structure, functionality, usage, and any specific requirements. They serve as a reference for other developers who may work on or interact with your project. Well-documented projects are easier to maintain, troubleshoot, and onboard new contributors.
Remember, effective documentation is a valuable asset that enhances the usability and longevity of your Node.js project.
Optimize and Secure
Optimizing and securing your Node.js project is essential for performance and protection. Optimization involves improving code efficiency, implementing caching mechanisms, and handling asynchronous operations effectively. This ensures your project runs smoothly and responds quickly to user requests.
Security measures include implementing input validation, authentication, and encryption to safeguard against common vulnerabilities and protect sensitive data. By optimizing and securing your Node.js project, you enhance its performance, user experience, and protect it from potential threats.
Deploy Your Project
Deploying your Node.js project is the final step in making it available to users in a production environment. Here’s an overview of the deployment process:
Prepare for Deployment
Before deploying, ensure your code is ready for production. Optimize its performance, handle errors, and make sure all dependencies are properly configured.
Choose a Hosting Provider
Select a hosting provider that suits your project’s needs. Options include cloud platforms like AWS, Azure, or Heroku, or traditional web hosting services. Consider factors like scalability, pricing, and ease of use.
Set up the Server
Configure the server environment where your Node.js application will run. This involves installing Node.js on the server, setting up necessary dependencies, and securing the environment.
Deploy Your Code
Upload your code to the server. This can be done through FTP, Git, or using deployment tools provided by your hosting provider. Ensure your code is placed in the correct directory and that all required files and folders are included.
Configure Environment Variables
Set environment variables to store sensitive information such as API keys or database credentials. These variables are read by your Node.js application, allowing you to keep sensitive data separate from your codebase.
Start the Application
Launch your Node.js application on the server using the appropriate command or script. This may involve using process managers like PM2 or systemd to ensure your application stays running in the background.
Test and Monitor
Perform thorough testing of your deployed application to ensure it functions as expected in the production environment. Set up monitoring tools to track performance, logs, and errors to proactively identify and address any issues.
Domain and DNS Configuration
If you have a custom domain, configure your DNS settings to point it to the server hosting your Node.js application. This allows users to access your application using your chosen domain name.
Continuous Deployment (Optional)
Consider setting up a continuous deployment workflow using tools like Jenkins, Travis CI, or GitHub Actions. This automates the deployment process whenever changes are pushed to your code repository.
Scale and Maintain
As your user base grows, monitor the performance of your application and scale resources accordingly. Regularly update dependencies, apply security patches, and keep your application up to date to ensure it remains secure and stable.
Deploying your Node.js project makes it accessible to users and allows you to gather real-world feedback. Remember to follow best practices, regularly backup your data, and keep an eye on security considerations to ensure a smooth and successful deployment.
Conclusion
In conclusion, Node.js development has revolutionized the way web applications are built and deployed. As a leading Node.js development company, we understand the power and versatility that Node.js brings to the table. With its event-driven, non-blocking I/O model, Node.js enables scalable and high-performance applications. Its vast ecosystem of modules and packages allows for rapid development and integration.
By leveraging the expertise of a Node.js development company, you can unlock the full potential of this technology and build robust, efficient, and feature-rich applications. Embrace the power of Node.js and propel your business to new heights with our professional Node.js development services.
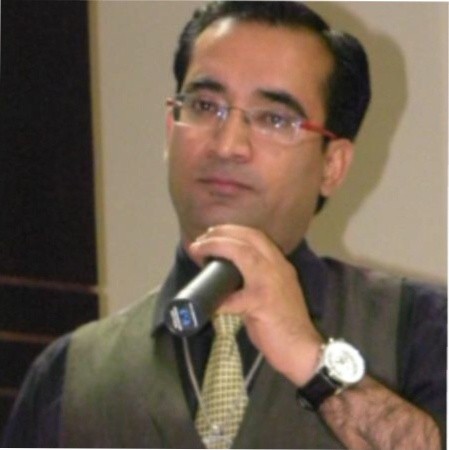
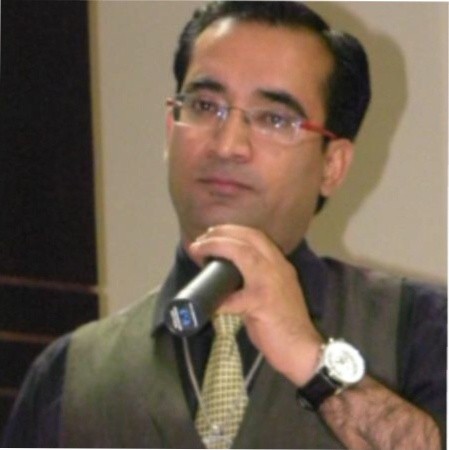
Naveen Khanna is the CEO of eBizneeds, a company renowned for its bespoke web and mobile app development. By delivering high-end modern solutions all over the globe, Naveen takes pleasure in sharing his rich experiences and views on emerging technological trends. He has worked in many domains, from education, entertainment, banking, manufacturing, healthcare, and real estate, sharing rich experience in delivering innovative solutions.