Last updated on December 22nd, 2023 at 07:15 am
In this comprehensive guide, we’ll delve deeper into the world of React Native, discover its capabilities, and understand how to create a better React app with native technology. React Native is a powerful framework that allows developers to create cross-platform mobile applications with ease. From its core principles to the benefits it offers, we’ll uncover everything you need to know about this cutting-edge technology.
Whether you’re a seasoned developer or just getting started, this guide will equip you with valuable insights to harness the potential of React Native and build outstanding apps.
Let’s embark on this journey of discovery into the realm of React Native and its endless possibilities.
What is React Native?
React Native is a JavaScript framework that allows developers to create mobile applications for Android, iOS, and other platforms using React, a popular JavaScript library for building user interfaces.
React Native combines the best parts of native development with React, enabling developers to create truly native apps that don’t compromise users’ experiences. It provides native platform-agnostic components such as View, Text, and Image that adhere to native UI elements.This means that your app uses the same native platform APIs as other apps do.
React Native lets you create cross-compatible mobile applications using only JavaScript, and you don’t have to worry about learning platform-specific application development languages such as Kotlin for Android or Swift for iOS.
Now you can use React Native in your existing Android and iOS projects or create a whole new app. With React Native, one team can maintain multiple platforms and share a common technology.
In summary, React Native is a powerful tool that allows developers to create mobile applications for multiple platforms using a single codebase. It is easy to learn and use, and it provides a seamless cross-platform development experience.
After discussing what is react native, let’s move onto its features.
Why React Native is Used For Mobile App Development?
React Native is widely used for mobile app development due to several key reasons:
Cross-platform compatibility
With React Native, developers can write code once and use it across both iOS and Android platforms. This saves significant development time and resources compared to building separate native apps for each platform.
Faster development
The ability to reuse code and components speeds up the development process. Changes can be made more efficiently, and updates can be rolled out quickly, leading to faster app development cycles.
Native-like performance
React Native bridges the gap between native components and the JavaScript code, resulting in a near-native user experience. The apps built with React Native feel responsive and perform as well as natively developed apps.
Cost-effective
Creating apps for multiple platforms with a single codebase reduces development costs. It allows businesses to reach a broader audience without the need for separate development teams or extensive resources.
Large community and ecosystem
React Native has a vast and active community of developers, providing extensive support, documentation, and a wide range of third-party libraries and packages. This ecosystem accelerates development and helps solve common challenges.
Hot Reload
React Native’s Hot Reload feature allows developers to see the changes made to the code in real-time on the simulator or the device. It significantly speeds up the debugging process and enhances productivity.
Backed by Facebook
React Native is an open-source project maintained by Facebook and has received continuous updates and improvements from a team of dedicated developers, ensuring its stability and reliability.
Due to these advantages, React Native has become a preferred choice for many developers and businesses looking to build mobile apps that are efficient, cost-effective, and provide a seamless user experience across platforms.
How Does React Native App Work?
React Native is a popular framework for building mobile apps that work seamlessly on both iOS and Android devices. It leverages the power of React, a JavaScript library for building user interfaces. Here’s a step-by-step explanation of how React Native apps work, accompanied by examples and images to make it easy to understand.
1. Development with JavaScript
React Native apps are primarily built using JavaScript, a widely-used programming language. Developers write code in JavaScript to define the app’s logic, design, and behavior.
2. React Components
React Native uses components, which are reusable building blocks for user interfaces. Developers create these components to define how different parts of the app should look and behave.
// Example of a React Native component
import React from ‘react’;
import { View, Text, StyleSheet } from ‘react-native’;
const AppHeader = () => {
return (
<View style={styles.header}>
<Text style={styles.text}>Welcome to My App</Text>
</View>
);
};
const styles = StyleSheet.create({
header: {
backgroundColor: ‘blue’,
padding: 20,
},
text: {
color: ‘white’,
fontSize: 24,
},
});
export default AppHeader;
3. Virtual DOM
React Native uses a Virtual DOM (Document Object Model) to efficiently update the user interface. It creates a virtual representation of the app’s UI in memory.
4. Native Modules
To interact with the device’s capabilities (e.g., camera, GPS), React Native uses native modules. These modules are written in native languages like Java (for Android) and Objective-C or Swift (for iOS).
5. JavaScript Bridge
The JavaScript Bridge is a critical component that connects the JavaScript code with the native modules. It allows JavaScript to communicate with the device’s native functionality.
6. Rendering on Device
The app’s UI is rendered on the device’s screen, combining the JavaScript-written components with the native elements. This process ensures the app looks and behaves natively.
7. Hot Reloading
React Native offers a feature called hot reloading, which allows developers to see the effects of code changes in real-time without restarting the app. This speeds up development and debugging.
8. Cross-Platform Compatibility
React Native enables code reusability across both iOS and Android platforms. Developers write code once and can deploy it to multiple platforms, saving time and effort.
9. Access to Native Features
React Native provides access to native features and libraries through JavaScript. Developers can use third-party libraries or create custom native modules to extend functionality.
10. Building and Deployment
After development, React Native apps can be built for iOS and Android separately. Developers use tools like Xcode and Android Studio to compile and package the app for distribution.

In summary, React Native simplifies mobile app development by allowing developers to use JavaScript to create a shared codebase for both iOS and Android. It bridges the gap between JavaScript code and native device capabilities, resulting in high-performance and native-looking apps.
This streamlined approach, combined with a rich ecosystem of libraries and tools, makes React Native a popular choice for mobile app development.
Features Required to Build React Native App
To create a React Native app, developers have access to a wide range of features that enhance productivity, efficiency, and the overall app-building experience. These features are part of the React Native ecosystem and simplify the process of building cross-platform mobile applications.
Here are some key features used to create React Native app:
Single Codebase
The fundamental advantage of React Native is its ability to use a single codebase for multiple platforms (iOS and Android). Developers write code in JavaScript and share it across both platforms, saving time and effort.
Hot Reload
React Native’s Hot Reload feature allows developers to see real-time changes made to the code without restarting the app. This significantly speeds up the development process and enhances productivity by providing immediate feedback.
Native Components
React Native utilizes native components to render the user interface. These components are equivalent to their native counterparts on iOS (UIKit) and Android (Android Views), ensuring a native-like user experience.
React Components
React Native allows developers to create reusable UI components using React, a popular JavaScript library for building user interfaces. These components can be composed together to form the app’s UI.
Third-Party Libraries
React Native has a vast ecosystem of third-party libraries and packages that can be easily integrated into the app. These libraries provide pre-built solutions for various functionalities, such as navigation, animations, and data management.
Platform-Specific Code
While most of the code is shared, React Native allows developers to write platform-specific code in Objective-C, Java, or Kotlin when necessary. This enables access to native APIs and functionalities unique to each platform.
Community Support
React Native has a large and active community of developers who contribute to its growth. This community provides extensive support, documentation, and solutions to common challenges, making the development process smoother.
Extensibility
Developers can extend React Native’s capabilities by creating custom native modules. These modules allow communication between JavaScript and native code, enabling access to device-specific features.
Performance Optimization
React Native optimizes performance by using a concept called “Thread Pooling,” where certain tasks are offloaded to separate threads. This ensures smooth and responsive app behavior.
Debugging Tools
React Native provides robust debugging tools that help developers identify and fix issues quickly. Tools like React DevTools and React Native Debugger aid in inspecting and debugging app components and state.
Benefits of React Native App
After talking about what is react native and its features, let’s move toward the benefits of React Native development and the reasons you must use it to create your mobile application.
More Affordable Than Native Development
If you use React Native, you need to create one JavaScript app. Contrarily, using a native approach requires starting from scratch and building separate iOS and Android applications.
With React Native, you only need to create one set of software. It means you can cut the cost of building and maintaining it in half while only requiring two teams of developers.
Third-Party Plugin Support
The fundamental React Native framework has lack of several elements.
It assures that developers can access third-party plugins, such as native and JavaScript modules, as compensation.
React native app also enables developers to link plugins to native or third-party modules, such as Google Maps, to integrate them into their projects.
Outstanding Performance
Responding native applications essentially function similarly to a native app created for the iOS or Android system.
Additionally, they are speedy due to the programming language’s optimization for mobile platforms.
React Native applications primarily utilize the Graphical Processing Unit rather than the CPU (GPU). They are thus much faster than hybrid technologies on several platforms.
Enhanced Reliability and Stability
React Native is crucial for making data binding simple. It also helps to safeguard parent data and guarantee that the child element does not change it.
React native also supports to maintain the stability and dependability of the applications. Before making a systematic upgrade to the product, developers should first alter their circumstances. Only the elements would be able to be modified during this experiment.
Moveable
App developers are not required to begin from scratch if they wish to switch the application to a different development platform in the long term.
React Native allows you to export the application to Xcode or react native android studio.
Using React Native for the creation of mobile apps has several advantages, and it also boosts the application’s adaptability.
JavaScript
According to a Statista investigation, global developers utilize JavaScript. 68% of survey respondents utilize JavaScript.
React developers don’t like Native since it’s written in JavaScript.
Enhancement to An Existing App
Do you currently have an app and want to upgrade it affordably?
Without modifying the program, we can add React Native UI elements.
It might be helpful when you merely wish to enhance an existing programme instead of entirely rebuilding it.
Which Applications Are Built with React Native?
Know about the several well-known applications which are created using React Native:
React Native is a widely used framework created by Facebook, which also used it to create its own ad management app for iOS and Android.
Instagram’s native app now uses the React Native framework. They started with WebView’s originally built Push notification view.
Skype
React Native with Skype entirely rebuilt the app, from the chat interface to the iconography. Additionally, they adjusted the desktop version to add to the mobile platform.
Pinterest is another app that uses React Native to provide a seamless cross-platform experience
Walmart
Walmart observed improvements like faster application performance, and simpler onboarding of additional teams after rewriting their app using React Native.
React Native Developer Tools
The top React Native tools are listed here to help developers start, grow, and finish the design process for all of their projects.
Atom
With Atom, you may edit documents on various operating systems, including Windows, Android, iOS, and Linux.
It has good themes, built-in package management, cross-platform editing, intellectual activity, and proofreading.
Selecting from various open-source programmes enables programmers to consider the functionality and features they want.
Nuclide
A highly personalized integrated development environment (IDE) tool called Nuclide helps programmers enhance project development.
Significant characteristics include working sets, rapid open, context view, a debugger, remote development, task runners, health statistics, etc.
They all combine to provide scalable and reliable solutions.
For development aid, it also provides auto-complete, inbuilt errors, and jump-to-definition capabilities. It has a strong development community and built-in help for problems.
Sublime Text
For React programming, Sublime Text is amazing option. It edits mark-up, code, and writing.
It has several community-developed plugins for programming and mark-up languages.
The tool provides developers with some functions to easily construct highly responsive cross-platform mobile apps.
It’s a Python API-enabled source code editor.
Visual Studio Code
It is a source code editor that supports the languages. It includes JavaScript, Go, Python, C++, and Fortran is called Visual Studio Code, sometimes abbreviated as VS Code.
This one is a better tools for providing fundamental functionality for popular programming languages.
These functionalities includes code folding, customizable snippets, syntax highlighting, and bracket matching. VS Code extensions include themes, languages, code analysis, and more.
Expo
Expo open-source framework lets React and JavaScript construct native applications for Web, Android, and iOS. A single codebase that works on several platforms helps development.
Other services it provides range from community forums and a Slack community to software applications, thorough documentation, and developer tools.
Expo’s accelerometer can react to variations in acceleration.
Expo may broadcast real-time updates and access APIs from devices running different operating systems.
Additionally, it can operate by serving, sharing, creating, and publishing concepts.
How Much Does It Cost to Develop a React Native App?
React native mobile apps often cost between $15,000 and $300,000.
The program’s entire cost might change based on its complexity, set of features, number of hours it took to build the code, developer hourly rates, distribution channels, etc.
Additionally, the location of a company that develops React Native mobile apps impacts the entire development cost.
Let’s check the breakdown of React Native App Development Costs.
React Native App Type |
Estimated Cost | Estimated Time Frame |
Simple |
$15,000 to $35,000 | 3-6 months |
Medium Complex | $35,000 to $80,000 |
6-9 months |
Complex | $80,000 to $300,000 |
9-12 months |
Factors Affecting React Native App Development Costs
Every step in creating an app demands a dedicated team and extensive preparation. The following features are mentioned with their estimated integration costs:
React native features |
Average Cost |
Login Interface |
$2,000-$4000 |
Admin Panel |
$4,000-$6,000 |
API Integrations |
$5,000- $5,500 |
Notification Panel |
$5,000-$7,500 |
Geolocation |
$15,000-$20,000 |
IoT Integration |
$25,000-$30,000 |
Streaming Portal |
$5,000-$10,000 |
E-Commerce |
$10,000-$15,000 |
Here is a list of the variables that influence the overall cost of developing a React Native app, ranging from selecting the special features to determining the size of the development team.
Let’s discuss in detail about what factors affecting react native development cost.
The App’s Complexity
Any app in stores may have low, medium, or high complexity. Six elements affect segmentation:
- Deployment Architecture Model: Custom or BaaS are the two choices for backend development. Clients choose their mobile app design with the Custom option, but with BaaS, they deal with a pre-made backend design.
- Admin Panel Development: Businesses may manage their applications, examine statistics, and alter content without React Native app developers using it. The complexity of the software increases with the admin panel’s feature richness.
- Third-Party Integration:Your app must interface with other functionalities to ease login and payment to be user-friendly. When constructing a React Native app as opposed to a native one, these connections are a little more complicated.
- In-App Purchase: Even though most apps accept in-app purchases, implementing this functionality is difficult. As a result, the more in-app purchase options you provide, the more complicated your React Native application
- Use of the Device’s In-Built Capabilities: Tablets and smartphones offer several functionalities. Bluetooth, GPS, Nearby barometers, and other tools may be connected to applications to enhance their functionality.
- Integration with the Business/Legacy System: Enterprise apps frequently require a local legacy system. Because they cannot function independently, these app categories, by default, fall into the medium-to-high complexity range.
User Authorization
Suppose you want to develop a mobility solution that requires user login or authorization.
In that case, the cost of developing an app using React Native will be slightly higher than without people signing up or joining.
App Category
Many factors change when we go from one app area to another, including the functionality available, security concerns, the number of actual users, etc.
The price of developing React Native apps increases due to their complexities.
For instance, a feature-rich mCommerce or on-demand application would cost significantly more than a standalone app with fewer features (like a timer or calculator).
Emphasis on Hardware
React native app cost more as you add hardware. Because, react Native is more complicated and more expensive to construct an IoT app than Native.
Do you want to know how much it costs to build IoT-based applications? Visit this post to learn more.
App Design
You need a solid concept design, a clear user flow, and scheduled transitions and transformations to keep users on your app as long as feasible.
However, creating displays and experiences that are certain to keep people’s attention costs money.
The design cost for mobile application in case of React Native app development is cheaper than Native app designing cost. Because there is need to design only one app version.
App’s Maintenance
There is little doubt that costs continue after the app is released, and you will need to update it often to meet customer expectations.
The user retention and participation levels for a customized app we created for Domino’s rose dramatically due to an app overhaul.
When we discuss app maintenance, we mainly refer to three distinct processes:
- App updates
- Changes to the design
- Bug fixes
The cost of maintaining an app is 20% of the cost of developing it with React Native.
Team Size
Three factors might affect the price of hiring React Native mobile app developers.
- Hire freelancers if you intend to do so
- To collaborate with a mid-cap firm,
- Whenever you intend to work with an organization of this size
The most economical option when using React Native for app development is to hire freelancers. If you worked with a big corporation, the beginning cost would be overpriced. Since, this is how they make money.
A mid-cap company whose primary hourly rate is between $30 and $50 is best. Because, they might be more open to a creative app idea than one with a high React Native app cost.
Agency’s Location
Agency’s Location React Native app cost vary depending on the location of the app development company.
For instance, choosing a React Native App Development Company in the US would cost you far more than in Eastern or Asian nations.
Here is a map showing the cost of React Native development per hour globally. You will have a good understanding of the price for React Native developers.
Add-Ons
Pricing depends significantly on custom options. You could wish to integrate it with other social media platforms or provide add-ons if you want to release a customer-focused application.
How To Create React Native App
After going through all the requirements for a practical app project, let’s dive into how to create react native app.
Conception and Idea
The first step is to come up with an idea for your mobile app. Think about the features, design, and purpose of the app. Consider how React Native app development can help you achieve your goals.
Research and Planning
Research the market to see if there’s a demand for your app idea. Plan the app’s functionality, user interface, and target audience. Create a rough sketch or wireframe of how you want the app to look.
Set up Development Environment
Install the necessary tools for React Native development. You’ll need Node.js, npm (Node Package Manager), and a code editor like Visual Studio Code.
Install React Native CLI
Use npm to install the React Native Command Line Interface (CLI). This tool will help you create and manage React Native projects.
Create a New Project
Use the React Native CLI to create a new project by running a simple command. This will set up the basic structure for your app.
Design User Interface
Use React Native’s components to design the user interface. You can create buttons, text inputs, and other UI elements that will make up your app’s screens.
Add Functionality
Write JavaScript code to add functionality to your app. You can handle user interactions, data fetching, and other app behaviors using React Native’s built-in features and third-party libraries.
Test Your App
Test your app on a simulator or a physical device to see how it behaves. Use React Native’s Hot Reload feature to make changes and instantly see them without rebuilding the entire app.
Debug and Refine
If you encounter any issues, use React Native’s debugging tools to identify and fix them. Continuously refine your app’s design and functionality based on user feedback.
Prepare for Release
Once your app is ready, prepare it for release. Create app icons, set up app store listings, and ensure all necessary information is provided.
App Store Submission
Follow the guidelines for submitting your app to the app stores (Apple App Store for iOS and Google Play Store for Android). This involves creating developer accounts, uploading app files, and completing the submission process.
Launch Your App
Once your app is approved, it will be available for download on the app stores. Promote your app on social media, websites, and other platforms to reach a wider audience.
Tech Stack Used to Create React Native App
Tech stacks are a rapidly growing industry, but what are they exactly?
The group of technologies that a react native development company uses to develop and oversee a project or programme is known as a “tech stack.”
This post will tell you about the most popular React tech stacks in 2022. And, it also discusses the advantages of utilizing React by cutting through the complexity and contextualizing ideas.
Next.js
It enables server-side processing and the creation of static webpages for React-based online apps.
The React framework, Next.js, provides the essential elements for web applications.
Next.js Platform handles the React-specific setup and infrastructure needs and gives your application more excellent structure, functionality, and optimizations.
The following are the primary commercial advantages of adopting Next.js:
- JS has created websites and apps that are adaptive to any screen size and work on every device.
- Customers may browse business websites or applications using their chosen instruments.
- Data security: Although the static site doesn’t directly relate to the databases, plugins, user information, or any other sensitive information, it is fully secure.
- Next’s increased availability of pre-built components has sped up the building of an MVP.
- You may instantly get feedback from real consumers and implement the required product modifications without wasting time or money.
Redux
Redux is among the most popular, modern, and cost-free frameworks for option panels for WordPress themes and plugins.
Because of WordPress’ flexibility, you may create any preferences and options for your project. Redux is a popular React state management framework.
Redux helps to address this problem and gives the developer the ability to keep the state of each element.
So, you can quickly find out the reason(s), time(s), and place(s) for a specific component change.
React-Query
React Query makes it easier for your React applications to request, cache, sync, and update server state.
The “missing data-fetching plugin” is sometimes called React.
React Query allows you to simplify several lines of cryptic code from your app with only a few characters of React Query logic.
Your application will be simple to maintain and expand without connecting different server-state data sources.
React Storybook
Storybook tests UI components in the development environment. It makes it possible for US developers to create and test separate components.
Project dependencies won’t impact component behaviour. Because, it also functions independently of the application.
You may automatically check your components graphically for errors using Storybook, record them, and reuse them later.
Storybook add-ons may alter responsive layouts or assess accessibility. Storybook integrates the vast majority of well-liked JavaScript UI frameworks.
React 18: Definition, Advantages and Updated Features
React 18 is the latest major version of the React JavaScript library for building user interfaces. It was released on March 29, 2022. React 18 is a major upgrade that can improve the performance, responsiveness, and accessibility of your React apps.
The Main New Features of React 18 Are:
- Concurrent rendering: This allows React to render multiple components at the same time, which can improve performance for applications with a lot of interactivity.
- Automatic batching: This groups together state changes that happen within a single event loop, which can also improve performance.
- Suspense improvements: This makes it easier to use Suspense to load lazy-loaded components and other resources.
- Streaming server rendering: This allows React to render the initial HTML of an application on the server before it is sent to the client, which can improve performance for SEO and first-load experiences.
- Transitions: This provides a way to animate changes to the UI, which can make applications more visually appealing.
In addition to these new features, React 18 also includes a number of other improvements, such as:
- Strict mode: This is a new mode that helps to prevent common errors and improve the performance of React applications.
- New hooks: This includes two new hooks, useIdand useDeferredValue, which can be used to improve the performance of React applications.
- Opt-in upgrading: This makes it easier to upgrade to React 18 without breaking existing applications.
Overall, React 18 is a significant release that brings a number of new features and improvements that can make React applications faster, more responsive, and more visually appealing.
Here Are Some of the Advantages of Using React 18:
- Improved performance: Concurrent rendering and automatic batching can improve the performance of React applications by reducing the amount of time it takes to render the UI.
- Enhanced interactivity: Concurrent rendering can also improve the interactivity of React applications by allowing them to respond to user input more quickly.
- Easier to use Suspense: The new Suspense improvements make it easier to use Suspense to load lazy-loaded components and other resources, which can improve the performance of React applications.
- Improved SEO: Streaming server rendering can improve the SEO of React applications by allowing search engines to crawl and index the initial HTML of the application.
- More visually appealing: Transitions can be used to animate changes to the UI, which can make React applications more visually appealing.
If you are building a React application, I recommend using React 18. It is the latest and greatest version of React, and it comes with a number of new features and improvements.
Conclusion
This blog has discussed what is react native in details.
It also elaborated that planning, effort, and money are required to turn your concept into finished software. Planning your app’s requirements and measures the growth of your potential market is the best course of action.
Before the app’s release, it is essential to get input from actual consumers who will use it. You might improve your app before deploying it using this strategy.
Start running ads as soon as the app is available to attract additional users.
After that, create a system to collect user feedback so you can keep improving your software. We hope this post has given you more knowledge about building React Native applications.
Why Should You Hire Us for React Native App Development?
Every small company should be able to take advantage of the digital age, extend their audience, provide digital apps, and web services, compete online with the big guys, and grow.
At eBizneeds, we think this is possible if you offer high-quality digital goods and services that give you the edge you need to grow and succeed.
In a competitive market, it will not succeed.
A service provider that shares your commitment to your business and is committed to its success. Hire developers who use React Native!
FAQs
What are the benefits of using React Native?
React Native offers advantages such as faster development, code reusability, cost-effectiveness, and a native-like performance and user experience on multiple platforms.
Is React Native suitable for all types of apps?
Yes, React Native is versatile and suitable for various app types, from simple to complex applications, including social media apps, e-commerce platforms, and more.
What are the core components of React Native?
React Native provides a set of built-in core components, including View, Text, Image, ScrollView, and others, which help in creating the user interface of the app.
Can I use native functionalities in a React Native app?
Yes, React Native allows developers to integrate platform-specific code (Objective-C, Java, or Kotlin) to access native APIs and features that are not available in the JavaScript environment.
How can I test my React Native app during development?
React Native provides tools like Expo and React Native CLI that allow you to test your app on simulators or physical devices. You can also use Hot Reload to see real-time changes while developing.
Is React Native well-supported by the developer community?
Yes, React Native has a large and active community of developers who contribute to its growth. The community provides extensive support, documentation, and third-party libraries to enhance the development process.
What are some popular apps built with React Native?
Many renowned apps use React Native, including Facebook, Instagram, Airbnb, UberEats, and Skype, showcasing its capability to power large-scale and successful applications.
How can I get started with React Native app development?
To get started, you need to set up a development environment, install React Native CLI, and create a new project. You can then design your app’s user interface, add functionality, and test it on simulators or devices before launching it on app stores.
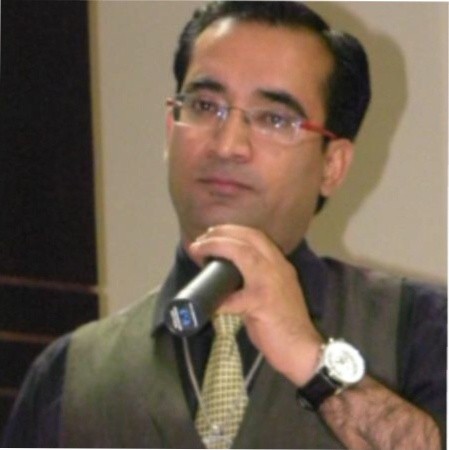
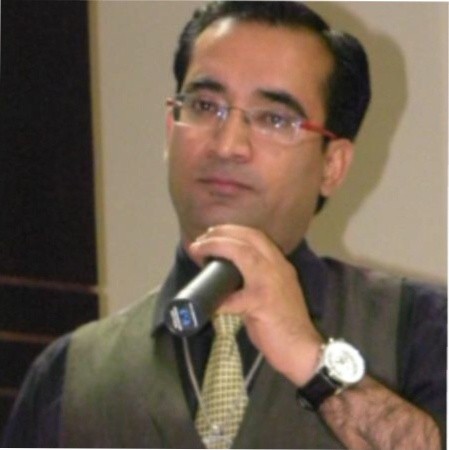
Naveen Khanna is the CEO of eBizneeds, a company renowned for its bespoke web and mobile app development. By delivering high-end modern solutions all over the globe, Naveen takes pleasure in sharing his rich experiences and views on emerging technological trends. He has worked in many domains, from education, entertainment, banking, manufacturing, healthcare, and real estate, sharing rich experience in delivering innovative solutions.